PostgreSQL for Django in Travis CI
December 5, 2016
With your Travis CI build, you can have access to a PostgreSQL server to run your tests.
Add a service in your .travis.yml
file for the database server like in the code block below:
services:
- postgresql
This will give you an instance of a PostgreSQL server available to you in your test environment.
Now you will need to create a database in your running database server. In your
.travis.yml
file add:
before_script:
- psql -c 'create database test_db;' -U postgres
This will create a database named test_db
by the user
postgres
. You can name your database anything you like, it is temporary
and used for this testing build.
By default when using PostgreSQL, the user is postgres
and no password.
The next thing to do is connect your Django application to the database server using your database credentials. We set the database name earlier in our travis yml file and we also know the default user and empty password. With this information we can set our credentials in two different ways.
To communicate information between your Django application and the database server, you will be using environment variables. These variables will hold the values of your database name and your database username.
Do not use your confidential production passwords and secret keys. You can create temporary information for your testing build. Travis CI makes a virtual machine for your tests and when finished it is destroyed, so make temporary credentials for your test builds. Do not use your confidential real secret key or passwords.
It is common to use evironment variables for this information, especially if you deploy to a service like Heroku.
In your Travis CI setting, you can set your environment variables. Here is an
example showing two environment variables SECRET_KEY
and the database
credentials DATABASE_URL
.
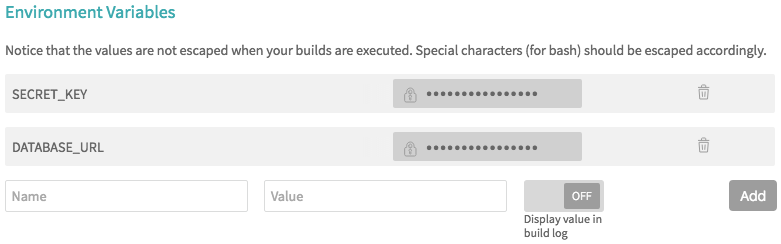
Two examples for setting database credentials.
Database URL
The database URL is commonly used is services like Heroku. For this reason it is my preferred way. My settings file is already configured for the URL, so I won’t need to change it. I have to set my ENV var and that is it.
To use the URL method you will need to import the dj_database_url
module into your Django settings file and define your database like this:
DATABASES = {'default': dj_database_url.config()}
The dj_database_url
module will extract the data from your URL and
create the needed database dictionary for Django.
With the above setting, you can define your database URL in your Travis CI environment variables like this:
DATABASE_URL = postgres://postgres@localhost/test_db
Note the username, no password, and database name from above.
Database Credentials
If you set your database, user name, and password in your Django settings file like this:
import os
"""
Django settings file for you project.
"""
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.postgresql',
'NAME': os.environ['DATABASE_NAME'],
'USER': os.environ['DATABASE_USER'],
'PASSWORD': os.environ['DATABASE_PASSWORD'],
}
}
You can set the above with environment variables in your Travis CI build settings. Using the default user for our postgres
database that is provided by Travis CI.
DATABASE_NAME = 'test_db'
DATABASE_USER = 'postgres'
DATABASE_PASSWORD = ''
Summary
In your .travis.yml
file, include a PostgreSQL database server and
create a test database. This will make available a database for your test
build.
In your Travis CI setting page, add your temporary environment variables for your database credentials.
Use the available environment variables in your Django settings file.