Bootstrap CSS in Django Forms
March 1, 2017
In this article I am going to show an example of adding Bootstrap CSS classes to an HTML form in a DJango project.
The Form
The form in this example will be an HTML form containing a select list (drop down menu) and a submit button. The select list will contain a list of U.S. States and the choices are generated by a function.
The Django form will be generated by implementing a Form class
.
Adding attributes to your form field is done with the form field’s widget
type.
Create a dictionary of attributes you would like to add to the form field and
assign it to the attrs
argument.
This example adds the Bootstrap class
of form-control
to the select list
field.
class StateSelectForm(forms.Form):
states = forms.ChoiceField(
choices=us_states(),
widget=forms.Select(attrs={'class': 'form-control'})
)
The CSS
In order to render a form, we will have to add the HTML form tags and submit button in our template. This allows us to add the necessary CSS classes to the form and any wrapping divs to contain the form. Adding Bootstrap classes to the submit button will be done in the template as well.
Add the Bootstrap classes as needed for each form field using the attrs
dictionary
described above.
<form action="" mehtod="post">
{% csrf_token %}
<div class="form-group">
{{ form.states }}
</div>
<input class="btn btn-primary" type="submit" value="Submit" />
</form>
Example Form Images
Without CSS
With Bootstrap CSS
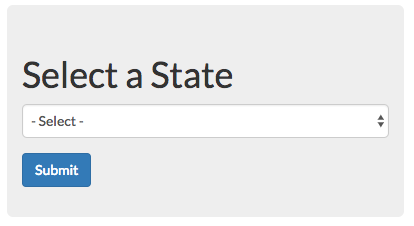
This example is a solution for adding a class to a simple form field. This is probably not the best, or most efficient, way to customize your form elements as it can be difficult with complext forms. Also including presentation implementations in the Python code logic is usually discouraged.
This approach may be acceptable for your particular application and allows adding other attributes to form fields. Sometimes adding dependencies, and the weight of large third-party apps, may not be warranted for a simple fix.
For more complex forms and more feature-rich solutions look into third-party Django applications: django-crispy-forms and django-widget-tweaks .
Summary
Add field specific attributes and classes using the field’s widget type. Add the required Bootstrap classes to the form and buttons in the HTML template. Don’t forget to include the reference to the Bootstrap CSS file in the HEAD of your HTML document.